How to Send Custom Push Notifications
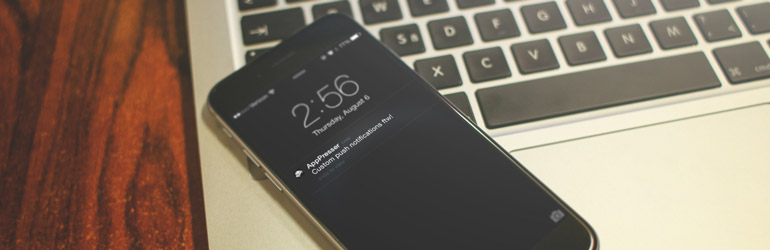
The AppPush extension allows you to send push notifications to your app through Pushwoosh or the WordPress dashboard.
Did you know you can also send custom push notifications based on almost any action in WordPress or in a plugin? There are some built-in integrations to send pushes when a new post is published, a private message is sent in BuddyPress, or manual sending.
If you want to send a push notification based on a different event in WordPress or a plugin, you can do that with the hooks and filters we will cover in this post.
Downloads
Here is a plugin that contains much of the sample push notifications code, ready for you to customize. It also enqueues a custom javascript file that helps you add custom ajax links, and shows you the proper way to load javascript in your app.
Download Custom AppPresser Plugin
Much of the code below is already in the downloads above, but it requires customization before you use it. Let’s look at what this code does, and how to modify it for your project.
Custom Push Notifications
Note: Requires AppPush 0.9.6+, and a paid Pushwoosh account with API access.
The apppush_send_notification allows you to send a notification based on any action. It looks like this:
function send_custom_push_notification( $data ) { apppush_send_notification( $data ); } add_action( 'yourhook', 'send_custom_push_notification');
With that function, you can send a notification based on any WordPress action, such as when an email is sent, or when a new comment is posted. Here’s an example of how to send a push notification when a new comment is posted:
function send_custom_push_notification( $id, $comment ) { $data = $comment->comment_content; if( function_exists('apppush_send_notification') ) { apppush_send_notification( $data ); } } add_action( 'wp_insert_comment', 'send_custom_push_notification', 10, 2 );
The $data variable is the text for the push message, in this case we made it the comment content.
Send Notifications Only to Specific Users
It’s also possible to send notifications only to certain devices, based on user ID.
For example, we use this in our BuddyPress integration to allow app users to send private messages to each other. You could also use this to subscribe users to segments, then send different notifications to different user groups.
Here’s an example:
// Change this hook add_action( 'save_post', 'push_to_devices' ); function push_to_devices() { if( !class_exists( 'AppPresser_Notifications' ) ) return; /* this should be an array of user IDs that you want to send the pushes to. AppPresser saves device IDs if the app user is a logged in member of your WordPress site, for example in BuddyPress. This will not work unless the user has logged in through your app. */ $recipients = array( 1, 24 ); $message = 'Hi there!'; $push = new AppPresser_Notifications_Update; $devices = $push->get_devices_by_user_id( $recipients ); if( $devices ) { $push->notification_send( 'now', $message, 1, $devices ); } }
In this example we are sending a notification that says “Hi there!” to users 1 and 24 when a post is saved. You can change the hook, message, and recipients to customize as needed.
Filtering Push Messages
You can filter all message content that comes from WordPress using the send_push_post_content filter. Example:
function send_custom_push( $message, $post_id, $post ) { if( 'apppush' === $post->post_type ) { $message = 'My custom push message'; } return $message; } add_filter( 'send_push_post_content', 'send_custom_push', 10, 3 );
The code above would override any message sent with the Notifications CPT in the WordPress Dashboard to say ‘My custom push message.’ You probably wouldn’t want to do that, but you can use this filter to do other stuff, like remove curse words, etc.
Note that all the code above only works with AppPush 0.9.6+ and a Pushwoosh account with API access.
Bonus: How to Properly Enqueue Javascript in AppPresser
Enqueuing a javascript file in an AppPresser app requires some special considerations.
Add a cordova-core dependency
To enqueue a javascript file in our app, but not on the desktop website, we have to add a dependency to cordova-core.
The code in our example plugin looks like this:
add_action( 'wp_enqueue_scripts', 'appp_custom_scripts' ); function appp_custom_scripts() { wp_enqueue_script( 'appp-custom', plugins_url( 'js/apppresser-custom.js' , __FILE__ ), array('jquery', 'cordova-core'), 1.0 ); }
You’ll notice we have cordova-core in our dependency array. That means the script won’t load unless we are in the app, it won’t load on the desktop.
Hook to load_ajax_content_done
The AppTheme uses ajax to load each page, which makes the app fast and smooth, with no page refreshes. Ajax loads differently than a normal page, so we have to make sure our scripts work on every page.
In the apppresser-custom.js file, you’ll see this at the bottom:
jQuery(document).ready( AppCustom.init ).on( 'load_ajax_content_done', AppCustom.init );
That means we run our code when the app loads, and every time a page is loaded via ajax.
You may be accustomed to loading javascript in the $(document).ready function, but we’ve also hooked to load_ajax_content_done. This is an event that fires in the AppTheme when a page loads via ajax, so it’s important that we hook to both.
The AppCustom.init function is an example of how to make a custom link load via ajax. This is useful to support custom plugins and any links that you want to load with ajax.
For further customization check out our docs, and let us know if there’s anything you’d like to see.
Can we send push notifications as image?
Can you setup ‘local push notifications’, where a user chooses to be reminded by the app at predetermined times?
Hi Jason, there is a local notification plugin for Phonegap, it’s pretty easy to use. You’d have to be on AppPresser 2, and then add the plugin and custom Javascript yourself, it’s not something we have a plugin for.
I have downloaded the plugin and activate it , tried to use the function “push_to_devices()” by uncomment the line :
add_action( ‘save_post’, ‘push_to_devices’, 999 );
and I just changed the array of user’s IDs , but it doesn’t work , it still sending notifications to everybody who downloaded the app , while I need to send notifications to the logged users only -or specific users- .
I wonder if there are missing steps ,or something I forgot to do ?
yes, we have same problem …
How would you send a push when a post is saved but only if it is a certain category? Thanks.