Using a child theme to customize your app
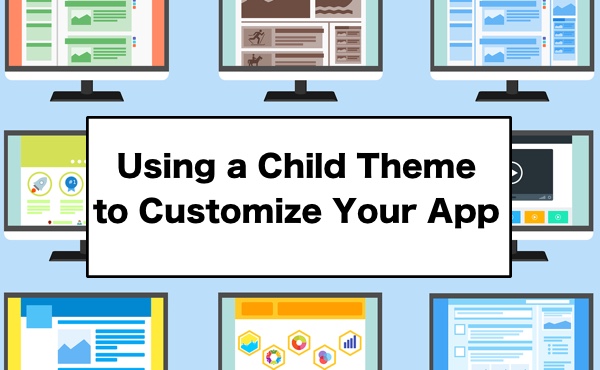
When building a WordPress website, using a child theme to customize your site’s look and functionality is considered a best practice.
It should come as no surprise that you should use a child theme when creating an app for your site. I briefly touched on this topic in my recent post where I provided an overview of the app building process. In today’s post, I’ll dive deeper into the process of using a child theme for your app.
Why use a child theme?
Using a child theme allows you to make changes to the look and functionality of your app, without losing those changes when the main theme is updated.
For the hotbookdeals.com website, I’m using one of the many child themes available for the Genesis theme framework. There are 3 main reasons why:
- Familiarity
I’ve built a number of sites using the Genesis framework. With each site build, I become more familiar with the built-in features of Genesis allowing me to build sites quickly. - Built-in Functionality
With Genesis, there are a ton of built-in features that make it easy to customize the look of the site without having to build every element from scratch. - Security and Feature Upgrades
Because I’m making all my theme customizations in a child theme, if an update becomes available for the Genesis framework, I can safely run the update without losing my edits.
These exact same principals applied when turning the site into an app. The only difference, instead of using Genesis, I used the Ion theme and created my own child theme.
The Ion theme, much like Genesis, has a ton of built-in features that make creating your app pretty simple. For starters, the header and menu functionality that will make your app look and feel like a professional app are already in there. You can also take a look at the list of Ionic Components that are built in and extremely easy to implement.
Creating a child theme
If you are new to working with WordPress, or you simply have never created a child theme before, I’m going to suggest you read this post from the WordPress codex. It will explain, in detail how to create your child theme. But for the rest of you, I’m going to assume you’ve created a child theme in your site’s theme folder and have both a style.css and functions.php file. Whenever I mention either of those file names moving forward, unless I say differently, I’m talking about the files that exist in your child theme folder.
Customizing the look
As I started building my app, I found that the Ion theme didn’t need to be changed much at all to give it the look I wanted. In the end, I needed to create a style to handle a larger featured image on the page that displayed the book details, plus I wanted to change the shape of the thumbnail image for the posts to fit the shape of the book cover. And the last elements I wanted to change were the widths of a couple border elements that were simply a personal preference. You can see the entirety of my style.css file below.
/* Theme Name: HBD App Theme URI: https://hotbookdeals.com/ Description: AppPresser theme for HBD Author: John Hawkins Author URI: http://vegasgeek.com Template: ion Version: 1.0.0 License: GNU General Public License v2 or later License URI: http://www.gnu.org/licenses/gpl-2.0.html Text Domain: hbdapp */ .single-deals .featured-image, .single-books .featured-image { float: left; height: 254px; margin: 15px 20px; width: 165px; } .home .post-list-item img { max-height: 120px; max-width: 80px; } .ion-theme .gform_wrapper .gsection { border-bottom: 3px solid #ccc; } .gform_body input[type="text"], .gform_body textarea { border: 1px solid #ccc; }
As you can see, there wasn’t a lot of customization needed. Between the Ion theme and the ability to tweak the colors in the Customizer, there wasn’t must custom work required to get the theme looking the way I wanted.
Customizing the functionality
When I built the website, I added all of the site specific functionality into a custom plugin. This means that, even when we’re developing for our app, we have access to all of that custom functionality. The end result being, in our child theme for the app, I only needed to add functionality that was specific to the app itself. I’ll break down the changes I made and additional files I created in order to customize my app.
functions.php
Other than enqueuing the style sheets, I only added two additional functions. Since the hotbookdeals website uses a custom post type called ‘deals’ for all of the listings, I wanted to change the default query and the search query both to focus solely on that CPT. This was handled by a couple quick pre_get_post calls as seen below.
// Have home page use deals CPT function hbd_use_deals_cpt( $query ) { if ( $query->is_home() && $query->is_main_query() ) { $query->set( 'post_type', 'deals' ); } } add_action( 'pre_get_posts', 'hbd_use_deals_cpt' ); // Limit search to deals CPT function hbd_app_limit_search_cpt( $query ) { if ( $query->is_search ) { $query->set( 'post_type', 'deals' ); }; return $query; }; add_filter('pre_get_posts','hbd_app_limit_search_cpt');
On the main page, where all of the current book deals are listed, I wanted to add the original price and the deal price to the listings. To do this, I copied the content-medialist.php file from the Ion theme folder over to my child theme folder and edited the content to display how I wanted.
<li id="post-<?php the_ID(); ?>" class="post-list-item"> <a class="item item-thumbnail-left item-text-wrap <?php if( class_exists( 'AppTransitions' ) ) { echo apply_filters('appp_transition_left', $classname ); } ?>" href="<?php the_permalink( $book_id ); ?>"><img src="<?php echo $book_thumb[0]; ?>"> <div class="item-title"><?php the_title(); ?></div> <div class="item-text"><?php echo $book_description; ?></div> <div class="item-text"> <span style="text-decoration:line-through">$<?php echo $book_price; ?></span> <strong><?php echo $deal_price; ?></strong> </div> </a> </li><!-- #post-## -->
When somebody clicks on one of the deal listings, there is a fair amount of data that needs to be presented. This meant I would need to customize that template file as well. To do that I started by copying single.php from the Ion theme and adding it to my child theme folder. I then renamed the file single-deals.php and began editing. In the code below, you can see that the first get_template_part call was edited to call a custom layout section called ‘singledeal’. Below that you’ll see that I commented out the get_template_part call because I don’t want to use it at the moment, but have some ideas for what may go there in the future.
get_header(); ?> <div id="content" class="site-content" role="main"> <?php while ( have_posts() ) : the_post(); ?> <?php get_template_part( 'content', 'singledeal' ); ?> <?php // get_template_part( 'content', 'below_post' ); ?> <?php endwhile; // end of the loop. ?> </div><!-- #content --> <?php get_footer(); ?>
For the custom layout section I mentioned, I made a copy of content-medialist.php from the ion theme folder into the child theme folder and renamed it content-singledeal.php. At the top of the file, I set up a lot of variables by pulling in post metadata (not pictured) so that I can lay out the display portion simply, like so. Note: Yes, there is some inline CSS styling that I intend to move to the style.css.
<article id="post-<?php the_ID(); ?>" <?php post_class(); ?>> <?php echo '<div class="featured-image">'; echo '<img src="'. $book_thumb[0] .'">'; echo '</div>'; ?> <div class="entry-content padding"> <h1 class="entry-title"><?php echo $title = apply_filters( 'appp_single_post_title', the_title(null,null,false) ); ?></h1> <p><span style="color:#888888;font-size:14px;display:block;line-height:1.1;">By <?php echo $author_list; ?></span></p> <p><span style="color:#a8210b;font-size:18px;font-weight:600;line-height:.5;font-style:italic;"><?php echo $bonus_text; ?></span></p> <p><span style="color:#000000;font-size:16px;display:block;line-height:1.2;"><?php echo $book_description; ?></span></p> <p><span style="color:#a8210b;font-size:26px;font-weight:600;line-height:1.1;padding-right:10px;">Deal Price: <?php echo $deal_price; ?></span> <p>Original Price: <span style="text-decoration:line-through">$<?php echo $book_price; ?></span> <p><span align="left" style="font-size:12px;line-height:3;text-align:center"> <span align="left" style="line-height:2.8!important"> <a href="<?php echo $amazon_link; ?>" style="background:#fb5f2a;border-radius:5px;color:#ffffff;display:inline-block;font-size:14px;font-weight:600;min-height:35px;line-height:2.5;margin:0;max-width:194px;padding:0;text-align:center;text-decoration:none;white-space:nowrap;width:100%" target="_blank">Buy at Amazon</a> <span style="font-size:4px;line-height:1"> </span> </span> </p> <p><span style="color:#888888;font-size:12px;display:block;line-height:2">This deal was originally posted on <?php echo get_the_date( 'n/j/Y' ); ?> and may no longer be available. Be sure to double-check the price prior to purchasing.</span></p> <footer class="entry-meta"> </footer><!-- .entry-meta --> </div><!-- .entry-content --> </article><!-- #post-## -->
The final modification I made was in footer.php. Again, I started by copying the file from the Ion theme folder into the child theme folder so that I could remove the login form since it wasn’t needed for the app.
That’s a wrap
Even though this is a deep dive into a specific site and subsequent app, the goal was to show you what’s possible with only a small amount of work. By now you probably already have a thousand ideas bouncing around your brain on how to turn your site into an app. We’ve created an Ion child theme to help you get started, and we can’t wait to see what you build with it! Go make it happen!
John,
Could you post an update that walks us through how this is done with AppPresser 3? I am having trouble getting AppPresser to use the child theme. Thanks!
Hi Scott
Is there an option to do this with AppPresser 3? It looks like the Ion theme needs to be activated first which can’t be done of course.
Yes, you don’t need to activate the Ion theme: https://docs.apppresser.com/article/337-child-theme